Have you ever wanted to create mesmerizing and realistic images based on your text prompts? With Stable Diffusion, you can generate stunning images that bring your imagination to life. In this article, we will explore how to use Stable Diffusion to generate high-quality images and unleash your creativity.
Getting Started
To begin, we need to set up the necessary dependencies. Open your terminal or command prompt and run the following commands to install the required packages:
!pip install diffusers torch torchvision
!pip install transformers
!pip install accelerate
These packages provide the tools and libraries needed for image generation and deep learning.
Step 1: Import the Required Packages
Let's start by importing the necessary packages. This includes diffusers
for Stable Diffusion, torch
for deep learning, torchvision
for image processing, transformers
for text processing, and accelerate
for GPU acceleration. Below is the code snippet for importing the packages:
from diffusers import StableDiffusionPipeline, EulerDiscreteScheduler
import torch
from IPython.display import Image, display
Step 2: Set Up Stable Diffusion Pipeline
Next, we need to set up the Stable Diffusion pipeline. This involves loading the pre-trained Stable Diffusion model and configuring the scheduler. Here's the code for setting up the pipeline:
model_id = "stabilityai/stable-diffusion-2-1-base"
scheduler = EulerDiscreteScheduler.from_pretrained(model_id, subfolder="scheduler")
pipe = StableDiffusionPipeline.from_pretrained(model_id, scheduler=scheduler, torch_dtype=torch.float16)
pipe = pipe.to("cuda")
Step 3: Generate an Image
With the pipeline set up, we can now generate an image based on a text prompt. In this step, we provide a prompt and use the pipeline to generate the image. Here's the code for generating the image:
prompt = "little astronaut standing on mars"
image = pipe(prompt).images[0]
Step 4: Save and Display the Image
Once the image is generated, we can save it to a file and display it. The following code snippet saves the image and displays it using the display
function from IPython.display
:
image_path = prompt + '.png'
image.save(image_path)
display(Image(filename=image_path))
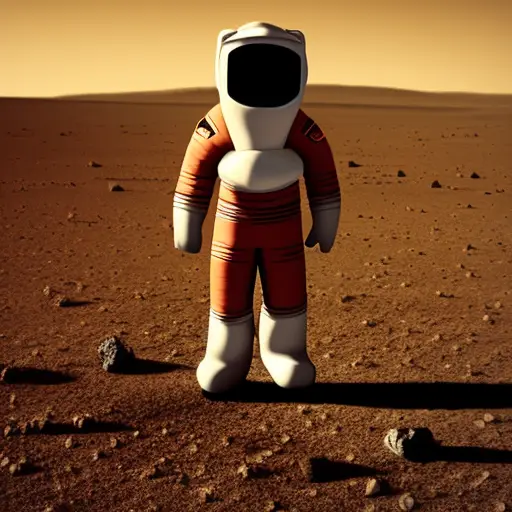
little astronaut standing on mars
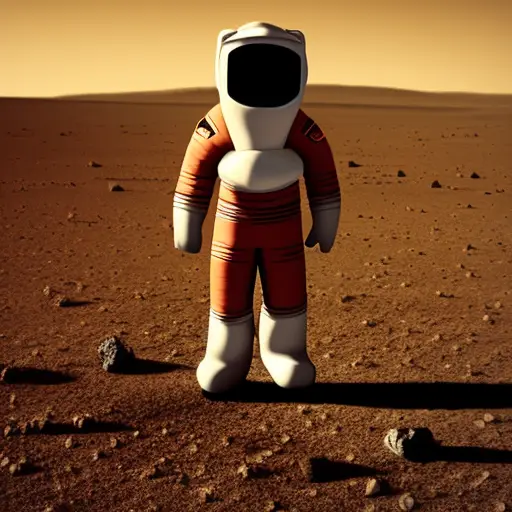
little astronaut standing on mars
Accelerating Image Generation
To speed up the image generation process, it is recommended to run the code on a GPU. The pipe.to("cuda")
line in the code snippet moves the pipeline to the GPU. If you don't have a GPU available, you can still run the code on a CPU, but keep in mind that it may take significantly longer, around 20 minutes, to generate a single image.
Conclusion
With Stable Diffusion, you now have the power to create captivating and realistic images based on your text prompts. By leveraging deep learning techniques, you can unlock endless possibilities for artistic expression and storytelling. Experiment with different prompts, explore various parameters, and let your imagination soar as you generate stunning images with Stable Diffusion.
Add a Comment: